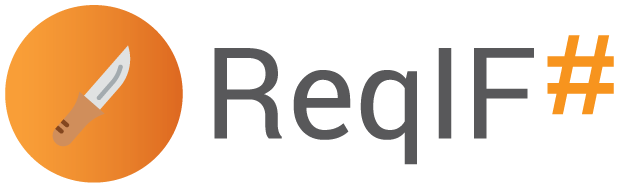
Introduction
A ReqIF document is an XML document that is structured according to the OMG ReqIF specification and is used for the exchange of requirements that have been authored in different tools such as CDP4-COMET, IBM Rational DOORS and many others. The ReqIF specification includes the ReqIF XML schema. The ReqIF standard makes use of certain XHTML modules that provide formatting for text such as tabular data, bold, italics etc. The ReqIFSharp library can be used with and without XML Schema validation. ReqIFSharp targets netstandard 2.0.
The two interfaces that are used the most in the reqifharp library are the
IReqIFDeserializer
interface and the
IReqIFSerializer
interface. The
IReqIFDeserializer
interface is used to deserialze a ReqIF document and create a
ReqIF
obejcts, while the
IReqIFSerializer
is used to serialize
ReqIF
objects.
Deserialization
An existing ReqIF document can be deserialized to an object graph using the
ReqIFDeserializer
class that implements the
IReqIFDeserializer
interface. The
IReqIFDeserializer
interface exposes one method, the
Deserialize
method. The
Deserialize
method returns an
IEnumerable{ReqIF}
. A ReqIF
object is the root node of a ReqIF document. The following code snippet
demonstrates how to deserialize
a ReqIF document without XML schema validation.
public void Deserialize()
{
var path = "some-file-path.reqif";
var deserializer = new ReqIFDeserializer();
var reqif = deserializer.Deserialize(path).First();
var header = reqif.TheHeader;
var content = reqif.CoreContent;
}
The following code snippet demonstrates how to deserialize using XML schema validation.
public void Deserialize()
{
var path = "some-file-path.reqif";
var deserializer = new ReqIFDeserializer();
var reqif = deserializer.Deserialize(path, true, this.ValidationEventHandler).First();
var header = reqif.TheHeader;
var content = reqif.CoreContent;
}
public void ValidationEventHandler(object sender, ValidationEventArgs validationEventArgs)
{
throw validationEventArgs.Exception;
}
Serialization
The
ReqIFSerializer
class that implements the
IReqIFSerializer
interface is used to serialize a requirements specification to a reqif
document. An
ReqIF
object, and it's content, can be constructed using the ReqIFSharp library. The
following snippet demonstrates
how this can be achieved.
public void Serialize()
{
var reqif = new ReqIF();
reqIF.Lang = "en";
var header = new ReqIFHeader()
{
Comment = "this is a comment",
CreationTime = DateTime.Parse("2015-12-01"),
Identifier = "reqifheader",
RepositoryId = "a repos id",
ReqIFToolId = "reqifsharp",
ReqIFVersion = "1.0",
SourceToolId = "reqifsharp",
Title = "this is a title"
};
this.reqIF.TheHeader = header;
var reqIfContent = new ReqIFContent();
this.reqIF.CoreContent = reqIfContent;
var reqifs = new List { reqif };
var resultFileUri = "some-file-path.reqif";
var serializer = new ReqIFSerializer(false);
serializer.Serialize(reqifs, resultFileUri , null);
}
For a more detailed explanation of the
ReqIFContent
of a
ReqIF
object please refer to the online
documentation.